Detecting Motion
Application areas such as security systems, automation, and robotics heavily rely on motion detection systems. The scope and depth of each system vary from one application area to another. However, the concept is the same. At the center of each motion detection system lies a motion sensor. This device samples the environment for different parameters and sends a measurement of the physical quantity to a computer. The computer then decides the presence or absence of motion in the environment.
There are different types of motion sensors, but we will focus mainly on the PIR sensor in this tutorial. We will describe how the devices work and the steps to connect the sensor to our Raspberry Pi. Finally, we will show how to activate a relay when the PIR sensor detects motion.
What is a PIR sensor
PIR sensors are devices that are used to detect motion within the sensor’s field of view. The full name is Passive Infrared, and in some texts, they are often referred to as Pyroelectric sensors. As the name suggests, the devices are passive sensors, which means that the sensor does not use its energy for detecting purposes. They work by detecting the energy radiated from the physical environment. Specifically, the module consists of a pyroelectric sensor. This is an electrically polarized crystal that generates a surface electric charge when exposed to infrared radiation levels. Humans and other animals such as cats and dogs are sources of infrared radiation, making the PIR sensor a perfect candidate for human or animal motion detection within the sensor’s range.
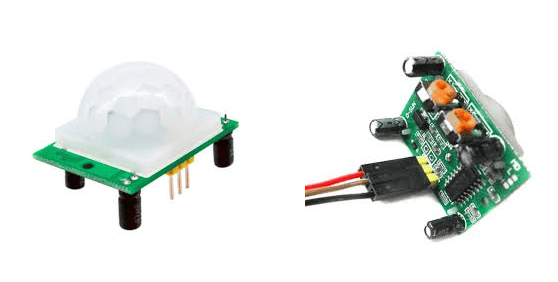
The white dome cover of the PIR sensor is known as the Fresnel Lens. This small device focuses the infrared signals onto the pyroelectric sensor, which means that the module can detect weak signals from the radiating sources. Also, a fresnel lens extends the sensor’s field of view.
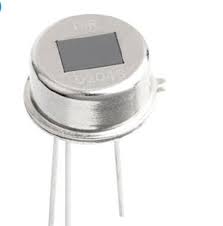
How PIR sensors work
Motion Detection
A PIR sensor, shown above, consists of crystals that respond to changes in infrared energy. The sensor itself is split into two windows, window A and window B, as shown in the diagram below. The two windows, A and B, are oriented in the same plane, and they have the same field of view, but they do not detect the IR levels simultaneously. There is a delay of a few microseconds between them, and this delay enables us to measure the IR difference between the two windows. This difference will let us know if the target has moved.
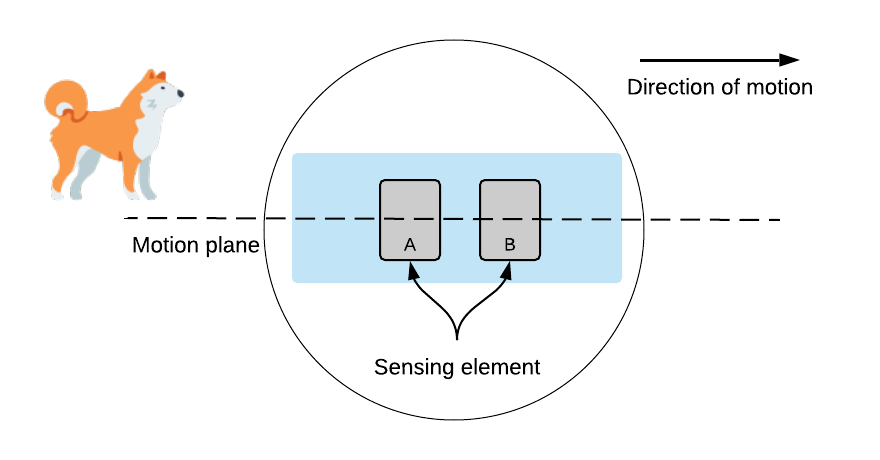
When the environment is empty, the two windows detect the same infrared levels and cancel each other out. However, when a warm target, such as a dog or human being, moves into the sensor’s field of view, the first window intercepts the IR. This causes a positive differential change between the two windows because the IR level in A will be higher than the IR level in B. When the object is leaving the area, the IR level in B will be higher than the IR in A, which causes a negative differential change between the two windows. Finally, the onboard IR sensor IC detects these pulse changes and decides motion in the environment, hence the presence of a target.
PIR Sensing Device
Pin Diagram
The complete PIR sensing device consists of the pyroelectric sensor and some supporting electronics. At the center of this lies the main chip—the BISS0001high-performance micropower PIR detector. The IC’s main job is to perform some processing on the received signals and output a pulse for further processing by the host CPU. Here is the description of the main components of the PIR sensor electronics hardware.
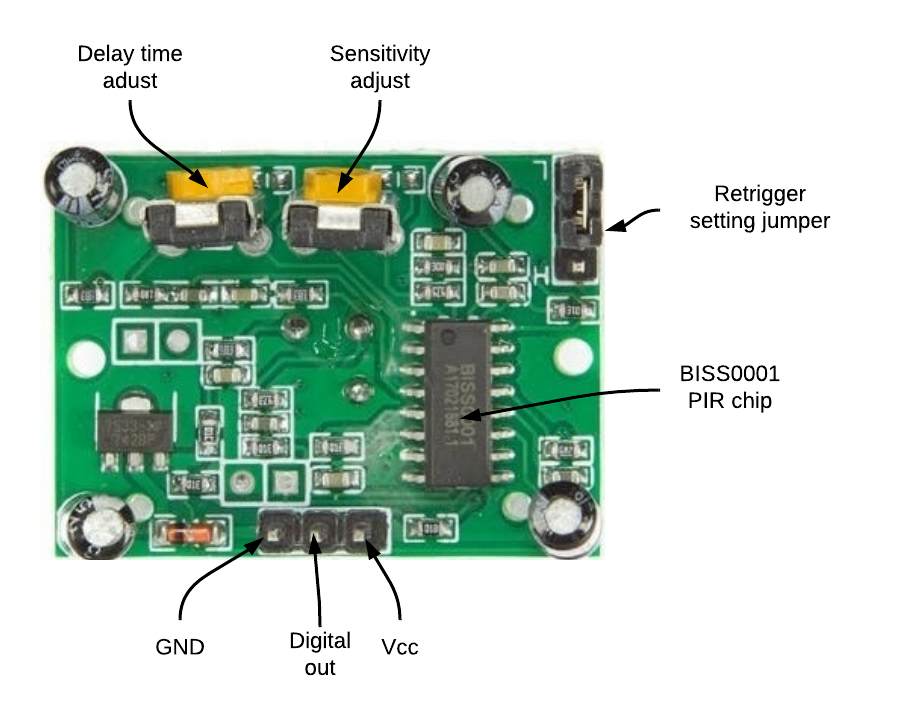
- The module has three pins: Ground and Vcc for powering the module. The Digital Out Pin gives a high logic level as a target moves within the sensor’s range.
- Delay time adjust. The first potentiometer adjusts the delay time. This is the time the output signal stays HIGH if the PIR detects motion. This time can range from 0.3 seconds to 5 minutes. Turning the potentiometer clockwise or right increases the delay time. Consecutively, turning the potentiometer counterclockwise or left decreases the delay time.
- Sensitivity adjust. The second potentiometer on the board is the sensitivity adjustment. We use this potentiometer to control the sensing range, which is approximately 3-7m. Turning this pot clockwise or right decreases the range, while turning it counterclockwise or left increases the sensing range.
- Trigger settings. There are two operational modes:
- Single trigger or non-repeatable mode: Here, when the sensor output is high and the delay time is over, the output will change from a HIGH to a LOW level.
- Repeatable trigger mode: This mode will keep the output high all the time until the detected object moves out of the sensor’s range.
Using an HC-SR501 PIR Sensor
How to connect a PIR sensor to the Rpi
The PIR sensor has three pins: GND, Vcc, and signal out. We connect these pins to the RPi, as shown in the diagram below.
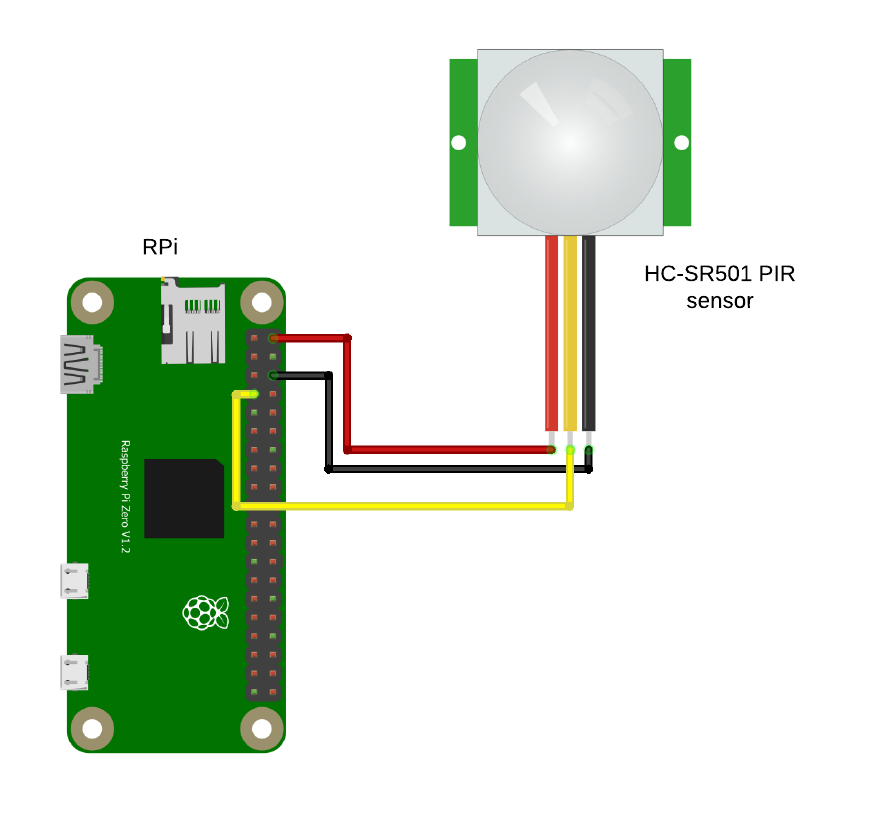
Programming the PIR sensor with Python
We are going to use the gpiozero
MotionSensor
class to read data from the module. Here is a starter code to interface the PIR sensor with the Raspberry Pi. If you do not have the gpiozero library installed, it’s time to do so now. Just use the command sudo apt install python3-gpiozero
, and you are good to go.
from gpiozero import MotionSensor pir = MotionSensor(4) while True: pir.wait_for_motion() print("Motion detected!")
The gpiozero MotionSensor library makes it easy to develop sensor applications on the RPi. This library has several functions such as waiting for a motion, what to do when the sensor detects motion, and what to do when no motion is detected. For our motion detection system, we import the MotionSensor library using the code: from gpiozero import MotionSensor
. We use the digital pin 4 for reading data from the PIR sensor: pir = MotionSensor(4)
. Then, we enter an infinite loop that waits for the presence of motion within the sensor’s range using pir.wait_for_motion()
. Finally, we take action when the PIR sensor’s state changes. In this case, we are only printing out to the terminal that motion has been detected. Below is the output.
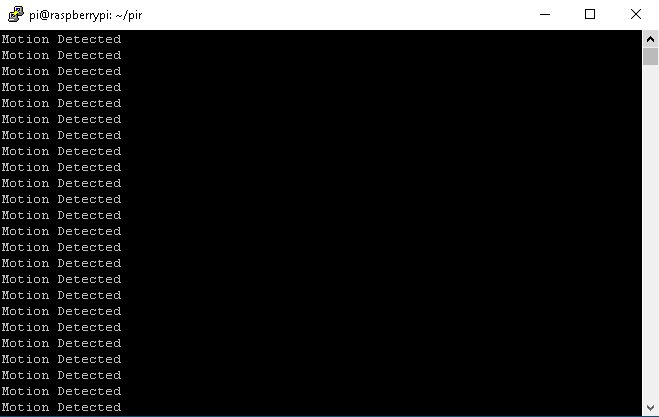
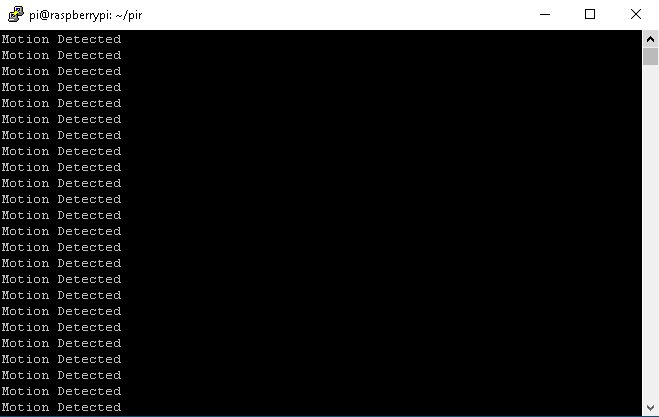
Sample Project
For this example project, we are going to activate a 5V relay when the PIR sensor detects motion in a room. We are going to need:
- Raspberry Pi
- HC-SR501 PIR sensor
- Relay module
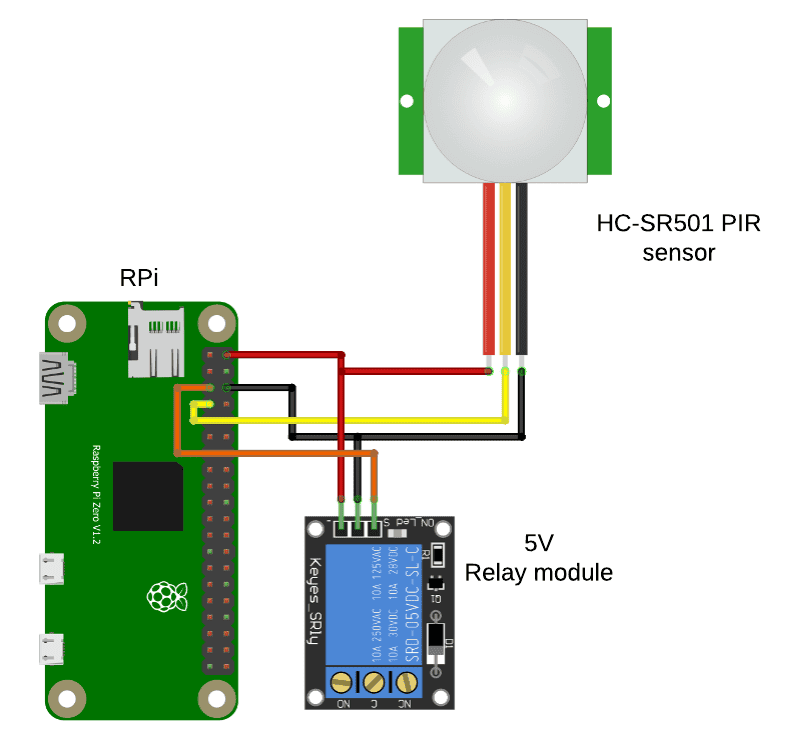
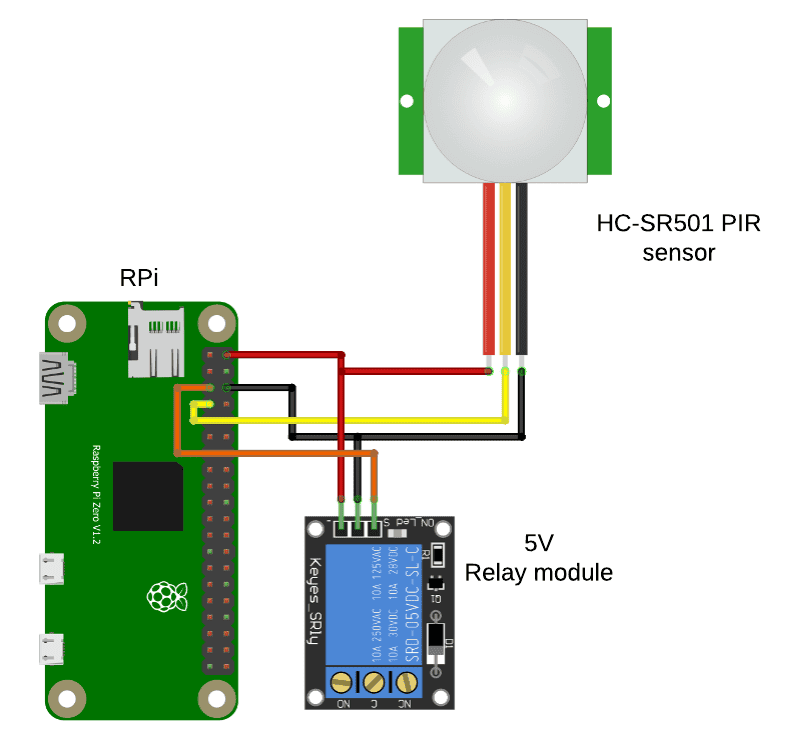
from gpiozero import MotionSensor, LED
from time import sleep pir = MotionSensor(4)
relay = LED(17) while True: pir.when_motion = relay.on pir.when_no_motion = relay.off
Code Description
The code above will activate a relay when the PIR sensor detects motion. We follow the same setup as we did before, but for this example, we are going to import an additional library from gpiozero. We import the LED library and attach an instance of the LED to pin 17 of the RPi. This will act as our output pin: relay = LED(17). The input pin of the motion sensor remains unchanged on pin 4: pir = MotionSensor(4)
. The LED library will also help us to change the state of the output pin when the sensor detects motion: pir.when_motion = relay.on
. To make sure that the output remains LOW when we do not have motion, we use: pir.when_no_motion = relay.off
. Finally, schedule the Python script with cron for running soon after boot. You can refer to this article for scheduling tasks with cron.
Hardware Connection


Please note that you will need to follow the best practices for mounting motion sensors to achieve the best PIR detection performance. That material is beyond the scope of this article.